VHDL Logical Operators and Signal Assignments for Combinational Logic
In this post, we discuss the VHDL logical operators, when-else statements , with-select statements and instantiation . These basic techniques allow us to model simple digital circuits.
In a previous post in this series, we looked at the way we use the VHDL entity, architecture and library keywords. These are important concepts which provide structure to our code and allow us to define the inputs and outputs of a component.
However, we can't do anything more than define inputs and outputs using this technique. In order to model digital circuits in VHDL, we need to take a closer look at the syntax of the language.
There are two main classes of digital circuit we can model in VHDL – combinational and sequential .
Combinational logic is the simplest of the two, consisting primarily of basic logic gates , such as ANDs, ORs and NOTs. When the circuit input changes, the output changes almost immediately (there is a small delay as signals propagate through the circuit).
Sequential circuits use a clock and require storage elements such as flip flops . As a result, changes in the output are synchronised to the circuit clock and are not immediate. We talk more specifically about modelling combinational logic in this post, whilst sequential logic is discussed in the next post.

Combinational Logic
The simplest elements to model in VHDL are the basic logic gates – AND, OR, NOR, NAND, NOT and XOR.
Each of these type of gates has a corresponding operator which implements their functionality. Collectively, these are known as logical operators in VHDL.
To demonstrate this concept, let us consider a simple two input AND gate such as that shown below.
The VHDL code shown below uses one of the logical operators to implement this basic circuit.
Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals. This is roughly equivalent to the = operator in most other programming languages.
In addition to signals, we can also define variables which we use inside of processes. In this case, we would have to use a different assignment operator (:=).
It is not important to understand variables in any detail to model combinational logic but we talk about them in the post on the VHDL process block .
The type of signal used is another important consideration. We talked about the most basic and common VHDL data types in a previous post.
As they represent some quantity or number, types such as real, time or integer are known as scalar types. We can't use the VHDL logical operators with these types and we most commonly use them with std_logic or std_logic_vectors.
Despite these considerations, this code example demonstrates how simple it is to model basic logic gates.
We can change the functionality of this circuit by replacing the AND operator with one of the other VHDL logical operators.
As an example, the VHDL code below models a three input XOR gate.
The NOT operator is slightly different to the other VHDL logical operators as it only has one input. The code snippet below shows the basic syntax for a NOT gate.
- Mixing VHDL Logical Operators
Combinational logic circuits almost always feature more than one type of gate. As a result of this, VHDL allows us to mix logical operators in order to create models of more complex circuits.
To demonstrate this concept, let’s consider a circuit featuring an AND gate and an OR gate. The circuit diagram below shows this circuit.
The code below shows the implementation of this circuit using VHDL.
This code should be easy to understand as it makes use of the logical operators we have already talked about. However, it is important to use brackets when modelling circuits with multiple logic gates, as shown in the above example. Not only does this ensure that the design works as intended, it also makes the intention of the code easier to understand.
- Reduction Functions
We can also use the logical operators on vector types in order to reduce them to a single bit. This is a useful feature as we can determine when all the bits in a vector are either 1 or 0.
We commonly do this for counters where we may want to know when the count reaches its maximum or minimum value.
The logical reduction functions were only introduced in VHDL-2008. Therefore, we can not use the logical operators to reduce vector types to a single bit when working with earlier standards.
The code snippet below shows the most common use cases for the VHDL reduction functions.
Mulitplexors in VHDL
In addition to logic gates, we often use multiplexors (mux for short) in combinational digital circuits. In VHDL, there are two different concurrent statements which we can use to model a mux.
The VHDL with select statement, also commonly referred to as selected signal assignment, is one of these constructs.
The other method we can use to concurrently model a mux is the VHDL when else statement.
In addition to this, we can also use a case statement to model a mux in VHDL . However, we talk about this in more detail in a later post as this method also requires us to have an understanding of the VHDL process block .
Let's look at the VHDL concurrent statements we can use to model a mux in more detail.
VHDL With Select Statement
When we use the with select statement in a VHDL design, we can assign different values to a signal based on the value of some other signal in our design.
The with select statement is probably the most intuitive way of modelling a mux in VHDL.
The code snippet below shows the basic syntax for the with select statement in VHDL.
When we use the VHDL with select statement, the <mux_out> field is assigned data based on the value of the <address> field.
When the <address> field is equal to <address1> then the <mux_out> signal is assigned to <a>, for example.
We use the the others clause at the end of the statement to capture instance when the address is a value other than those explicitly listed.
We can exclude the others clause if we explicitly list all of the possible input combinations.
- With Select Mux Example
Let’s consider a simple four to one multiplexer to give a practical example of the with select statement. The output Q is set to one of the four inputs (A,B, C or D) depending on the value of the addr input signal.
The circuit diagram below shows this circuit.
This circuit is simple to implement using the VHDL with select statement, as shown in the code snippet below.
VHDL When Else Statements
We use the when statement in VHDL to assign different values to a signal based on boolean expressions .
In this case, we actually write a different expression for each of the values which could be assigned to a signal. When one of these conditions evaluates as true, the signal is assigned the value associated with this condition.
The code snippet below shows the basic syntax for the VHDL when else statement.
When we use the when else statement in VHDL, the boolean expression is written after the when keyword. If this condition evaluates as true, then the <mux_out> field is assigned to the value stated before the relevant when keyword.
For example, if the <address> field in the above example is equal to <address1> then the value of <a> is assigned to <mux_out>.
When this condition evaluates as false, the next condition in the sequence is evaluated.
We use the else keyword to separate the different conditions and assignments in our code.
The final else statement captures the instances when the address is a value other than those explicitly listed. We only use this if we haven't explicitly listed all possible combinations of the <address> field.
- When Else Mux Example
Let’s consider the simple four to one multiplexer again in order to give a practical example of the when else statement in VHDL. The output Q is set to one of the four inputs (A,B, C or D) based on the value of the addr signal. This is exactly the same as the previous example we used for the with select statement.
The VHDL code shown below implements this circuit using the when else statement.
- Comparison of Mux Modelling Techniques in VHDL
When we write VHDL code, the with select and when else statements perform the same function. In addition, we will get the same synthesis results from both statements in almost all cases.
In a purely technical sense, there is no major advantage to using one over the other. The choice of which one to use is often a purely stylistic choice.
When we use the with select statement, we can only use a single signal to determine which data will get assigned.
This is in contrast to the when else statements which can also include logical descriptors.
This means we can often write more succinct VHDL code by using the when else statement. This is especially true when we need to use a logic circuit to drive the address bits.
Let's consider the circuit shown below as an example.
To model this using a using a with select statement in VHDL, we would need to write code which specifically models the AND gate.
We must then include the output of this code in the with select statement which models the multiplexer.
The code snippet below shows this implementation.
Although this code would function as needed, using a when else statement would give us more succinct code. Whilst this will have no impact on the way the device works, it is good practice to write clear code. This help to make the design more maintainable for anyone who has to modify it in the future.
The VHDL code snippet below shows the same circuit implemented with a when else statement.
Instantiating Components in VHDL
Up until this point, we have shown how we can use the VHDL language to describe the behavior of circuits.
However, we can also connect a number of previously defined VHDL entity architecture pairs in order to build a more complex circuit.
This is similar to connecting electronic components in a physical circuit.
There are two methods we can use for this in VHDL – component instantiation and direct entity instantiation .
- VHDL Component Instantiation
When using component instantiation in VHDL, we must define a component before it is used.
We can either do this before the main code, in the same way we would declare a signal, or in a separate package.
VHDL packages are similar to headers or libraries in other programming languages and we discuss these in a later post.
When writing VHDL, we declare a component using the syntax shown below. The component name and the ports must match the names in the original entity.
After declaring our component, we can instantiate it within an architecture using the syntax shown below. The <instance_name> must be unique for every instantiation within an architecture.
In VHDL, we use a port map to connect the ports of our component to signals in our architecture.
The signals which we use in our VHDL port map, such as <signal_name1> in the example above, must be declared before they can be used.
As VHDL is a strongly typed language, the signals we use in the port map must also match the type of the port they connect to.
When we write VHDL code, we may also wish to leave some ports unconnected.
For example, we may have a component which models the behaviour of a JK flip flop . However, we only need to use the inverted output in our design meaning. Therefore, we do not want to connect the non-inverted output to a signal in our architecture.
We can use the open keyword to indicate that we don't make a connection to one of the ports.
However, we can only use the open VHDL keyword for outputs.
If we attempt to leave inputs to our components open, our VHDL compiler will raise an error.
- VHDL Direct Entity Instantiation
The second instantiation technique is known as direct entity instantiation.
Using this method we can directly connect the entity in a new design without declaring a component first.
The code snippet below shows how we use direct entity instantiation in VHDL.
As with the component instantiation technique, <instance_name> must be unique for each instantiation in an architecture.
There are two extra requirements for this type of instantiation. We must explicitly state the name of both the library and the architecture which we want to use. This is shown in the example above by the <library_name> and <architecture_name> labels.
Once the component is instantiated within a VHDL architecture, we use a port map to connect signals to the ports. We use the VHDL port map in the same way for both direct entity and component instantiation.
Which types can not be used with the VHDL logical operators?
Scalar types such as integer and real.
Write the code for a 4 input NAND gate
We can use two different types of statement to model multiplexors in VHDL, what are they?
The with select statement and the when else statement
Write the code for an 8 input multiplexor using both types of statement
Write the code to instantiate a two input AND component using both direct entity and component instantiation. Assume that the AND gate is compiled in the work library and the architecture is named rtl.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Table of Contents
Sign up free for exclusive content.
Don't Miss Out
We are about to launch exclusive video content. Sign up to hear about it first.
- Product Manual
- Knowledge Base
- Release Notes
- Tech Articles
- Screencasts
Signal Assignments in VHDL: with/select, when/else and case
Sometimes, there is more than one way to do something in VHDL. OK, most of the time , you can do things in many ways in VHDL. Let’s look at the situation where you want to assign different values to a signal, based on the value of another signal.
With / Select
The most specific way to do this is with as selected signal assignment. Based on several possible values of a , you assign a value to b . No redundancy in the code here. The official name for this VHDL with/select assignment is the selected signal assignment .
When / Else Assignment
The construct of a conditional signal assignment is a little more general. For each option, you have to give a condition. This means that you could write any boolean expression as a condition, which give you more freedom than equality checking. While this construct would give you more freedom, there is a bit more redundancy too. We had to write the equality check ( a = ) on every line. If you use a signal with a long name, this will make your code bulkier. Also, the separator that’s used in the selected signal assignment was a comma. In the conditional signal assignment, you need the else keyword. More code for the same functionality. Official name for this VHDL when/else assignment is the conditional signal assignment
Combinational Process with Case Statement
The most generally usable construct is a process. Inside this process, you can write a case statement, or a cascade of if statements. There is even more redundancy here. You the skeleton code for a process (begin, end) and the sensitivity list. That’s not a big effort, but while I was drafting this, I had put b in the sensitivity list instead of a . Easy to make a small misstake. You also need to specify what happens in the other cases. Of course, you could do the same thing with a bunch of IF-statements, either consecutive or nested, but a case statement looks so much nicer.
While this last code snippet is the largest and perhaps most error-prone, it is probably also the most common. It uses two familiar and often-used constructs: the process and the case statements.
Hard to remember
The problem with the selected and conditional signal assignments is that there is no logic in their syntax. The meaning is almost identical, but the syntax is just different enough to throw you off. I know many engineers who permanenty have a copy of the Doulos Golden Reference Guide to VHDL lying on their desks. Which is good for Doulos, because their name gets mentioned all the time. But most people just memorize one way of getting the job done and stick with it.
- VHDL Pragmas (blog post)
- Records in VHDL: Initialization and Constraining unconstrained fields (blog post)
- Finite State Machine (FSM) encoding in VHDL: binary, one-hot, and others (blog post)
- "Use" and "Library" in VHDL (blog post)
- The scope of VHDL use clauses and VHDL library clauses (blog post)
- Network Sites:
- Technical Articles
- Market Insights

- Or sign in with
- iHeartRadio

Concurrent Conditional and Selected Signal Assignment in VHDL
Join our engineering community sign-in with:.
This article will review the concurrent signal assignment statements in VHDL.
This article will first review the concept of concurrency in hardware description languages. Then, it will discuss two concurrent signal assignment statements in VHDL: the selected signal assignment and the conditional signal assignment. After giving some examples, we will briefly compare these two types of signal assignment statements.
Please see my article introducing the concept of VHDL if you're not familiar with it.
Concurrent vs. Sequential Statements
To understand the difference between the concurrent statements and the sequential ones, let’s consider a simple combinational circuit as shown in Figure 1.
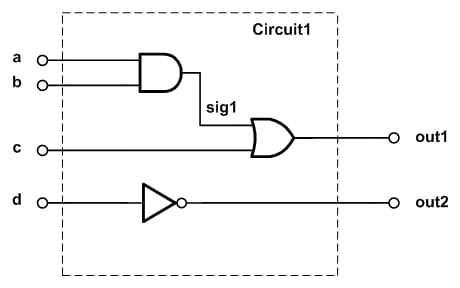
Figure 1. A combinational circuit.
If we consider the operation of the three logic gates of this figure, we observe that each gate processes its current input(s) in an independent manner from other gates. These physical components are operating simultaneously. The moment they are powered, they will “concurrently” fulfill their functionality. Note that while, in practice, the AND gate has a delay to produce a valid output, this does not mean that the OR gate will stop its functionality and wait until the output of the AND gate is produced. The OR gate will function all the time; however, its output will not be valid until its inputs have settled.
Now, let’s examine the VHDL description of Figure 1. This is shown below:
The main part that we are here interested in is the definition of the three gates:
Each of these lines describes a physical component in Figure 1. For example, the second line, which describes the OR gate, takes sig1 and c as inputs and produces the OR of these two values. We saw that the physical components of Figure 1 operate concurrently. Hence, it is reasonable to expect that the VHDL description of these gates should be evaluated in a concurrent manner. In other words, the above three lines of the code are executed at the same time and there is no significance to the order of these statements. As a result, we can rewrite the architecture section of the above code as below:
Since these statements are evaluated at the same time, we call them concurrent statements. This type of code is quite different from what we have learned in basic computer programming where the lines of code are executed one after the other. For example, consider the following MATLAB code:
This code produces out1=1 and out2=1 . However, if we change the order of the statements to the following, the program will stop working because we are trying to use sig1 before it is generated.
While the VHDL code describing Figure 1 was executed concurrently, the above MATLAB code is evaluated sequentially (i.e., one line after the other). VHDL supports both the concurrent statements and the sequential ones. It's clear that the concurrent VHDL statements will allow us to easily describe a circuit such as the one in Figure 1 above. In a future article, we'll see that the sequential VHDL statements allow us to have a safer description of sequential circuits. Furthermore, using the sequential VHDL, we can easily describe a digital circuit in a behavioral manner. This capability can significantly facilitate digital hardware design.
The following figure illustrates the difference between concurrent and sequential statements.

Figure 2. The difference between concurrent and sequential statements. Image courtesy of VHDL Made Easy .
Now let's take a look at two concurrent signal assignment statements in VHDL: “the selected signal assignment statement” and “the conditional signal assignment statement”.
Selected Signal Assignment or the “With/Select” Statement
Consider an n -to-one multiplexer as shown in Figure 3. This block should choose one out of its n inputs and transfer the value of this input to the output terminal, i.e., output_signal .
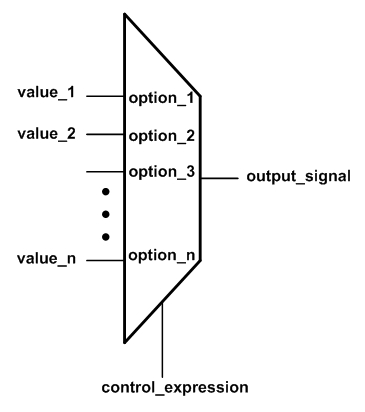
Figure 3. A multiplexer selects one of its n inputs based on the value of the control_expression.
The selected signal assignment allows us to implement the functionality of a multiplexer. For example, the VHDL code describing the multiplexer of Figure 3 will be
Here, the value of the control_expression will be compared with the n possible options, i.e., option_1 , option_2 , …, option_n . When a match is found, the value corresponding to that particular option will be assigned to the output signal, i.e., output_signal . For example, if control_expression is the same as option_2 , then value_2 will be assigned to the output_signal .
Note that the options of a “with/select” assignment must be mutually exclusive, i.e., one option cannot be used more than once. Moreover, all the possible values of the control_expression must be included in the set of the options. The following example clarifies these points.
Example 1 : Use the "with/select" statement to describe a one-bit 4-to-1 multiplexer. Assume that the inputs to be selected are a , b , c , and d . And, a two-bit signal, sel , is used to choose the desired input and assign it to out1 .
The code for this multiplexer is given below:
Note that since the std_logic data type can take values other than “0” and “1” , the last line of the “with/select” statement needs to use the keyword “ others ” to take all the possible values of sel into account.
The following figure shows the simulation of this code using the Xilinx ISE simulator. (In case you’re not familiar with ISE, see this tutorial .) As shown in this figure, from 0 nanosecond (ns) until 300 ns the select input, sel , is 00, and, hence, out1 follows the input a . Similarly, you can verify the intended operation for the rest of the simulation interval.

Figure 4. The ISE simulation for the multiplexer of Example 1.
Example 2 : Use the “with/select” statement to describe a 4-to-2 priority encoder with the truth table shown below.
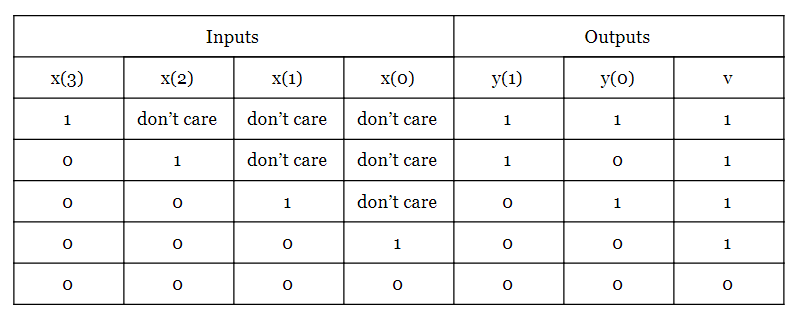
The following VHDL code can be used to describe the above truth table:
The ISE simulation is shown in Figure 5.

Figure 5. The ISE simulation for the priority encoder of Example 2.
Conditional signal assignment or the “when/else” statement.
The “when/else” statement is another way to describe the concurrent signal assignments similar to those in Examples 1 and 2. Since the syntax of this type of signal assignment is quite descriptive, let’s first see the VHDL code of a one-bit 4-to-1 multiplexer using the “when/else” statement and then discuss some details.
Example 3 : Use the when/else statement to describe a one-bit 4-to-1 multiplexer. Assume that the inputs to be selected are a , b , c , and d . And, a two-bit signal, sel , is used to choose the desired input and assign it to out1 .
The code will be
In this case, the expressions after “when” are evaluated successively until a true expression is found. The assignment corresponding to this true expression will be performed. If none of these expressions are true, the last assignment will be executed. In general, the syntax of the “when/else” statement will be:
We should emphasize that the expressions after the “when” clauses are evaluated successively. As a result, the expressions evaluated earlier has a higher priority compared to the next ones. Considering this, we can obtain the conceptual diagram of this assignment as shown in Figure 6. This figure illustrates a conditional signal assignment with three “when” clauses.

Figure 6. The conceptual implementation of a “when/else” statement with three “when” clauses.
Let’s review the main features of the selected signal assignment and the conditional signal assignment.
“With/Select” vs. “When/Else” Assignment
As mentioned above, the options of a “with/select” assignment must be mutually exclusive, i.e., one option cannot be used more than once. Moreover, all the possible values of the control_expression must be included in the set of options. While the “with/select” assignment has a common controlling expression, a “when/else” assignment can operate on expressions with different arguments. For example, consider the following lines of code:
In this case, the expressions are evaluating two different signals, i.e., reset1 and clk .
For the “when/else” assignment, we may or may not include all the possible values of the expressions to be evaluated. For example, the multiplexer of Example 3 covers all the possible values of sel ; however, the above code does not. The above code implies that out1 should retain its previous value when none of the expressions are true. This causes the inference of a latch in the synthesized circuit.
Another important difference between the “with/select” and “when/else” assignment can be seen by comparing the conceptual implementation of these two statements. The priority network of Figure 6 involves a cascade of several logic gates. However, the “with/select” assignment avoids this chain structure and has a balanced structure. As a result, in theory, the “with/select” statement may have better performance in terms of the delay and area (see RTL Hardware Design Using VHDL: Coding for Efficiency, Portability, and Scalability , Xilinx HDL Coding Hints , and Guide to HDL Coding Styles for Synthesis ).
In practice, we generally don’t see this difference because many synthesis software packages, such as the Xilinx XST, try not to infer a priority encoded logic. Though we can use the PRIORITY_EXTRACT constraint of XST to force priority encoder inference, Xilinx strongly suggests that we use this constraint on a signal-by-signal basis; otherwise, the constraint may guide us towards sub-optimal results. For more details see page 79 of the XST user guide .
- Concurrent statements are executed at the same time and there is no significance to the order of these statements. This type of code is quite different from what we have learned in basic computer programming where the lines of code are executed one after the other.
- The selected signal assignment or the "with/select" assignment allows us to implement the functionality of a multiplexer.
- The options of a “with/select” assignment must be mutually exclusive, i.e., one option cannot be used more than once. Moreover, all the possible values of the control_expression must be included in the set of the options.
- For the "when/else" statement, the expressions after the “when” clauses are evaluated successively. As a result, the expressions evaluated earlier has a higher priority compared to the next ones.
- One important difference between the “with/select” and “when/else” assignment can be seen by comparing the conceptual implementation of these two statements. The "when/else" statement has a priority network; however, the “with/select” assignment avoids this chain structure and has a balanced structure.
To see a complete list of my articles, please visit this page .
Featured image used courtesy of Parallella .
Related Content
- Safety in Sensing: Sensor Technology in Smart Cities
- Thermocouple Signal Conditioners and Signal Conditioning Near the Cold Junction
- Reducing Distortion in Tape Recordings with Hysteresis in SPICE
- Test & Measurement in Quantum Computing
- Open RAN – Network Performance in the Lab and in the Field
- Looking for Good Waves: The Importance of Signal Integrity in High-Speed PCB Design
Learn More About:
- programmable logic

Great content. The link to the ISE guide requires password. Can we get that posted again? Thanks!
You May Also Like

Minimum Size, Maximum Performance in AOTA Inductors
In Partnership with Future Electronics


Switch Roundup: New Devices Make Switches Smaller and Faster
by Aaron Carman

Renesas Unwraps MCU-Based Sensor Module for Smart Air Quality Monitoring
by Mike Falter

System Solution Guide: Machine Vision

AMD Quietly Drops New Ryzen Processors Without Integrated Graphics
by Jake Hertz

Welcome Back
Don't have an AAC account? Create one now .
Forgot your password? Click here .

Signal Assignment | LRM §8.4, §9.5. |
A signal assignment statement modifies the target signal
Description:
A Signal assignment statement can appear inside a process (sequential statement) or directly in an architecture (concurrent statement). The target signal can be either a name (simple, selected, indexed, or slice) or an aggregate .
A signal assignment with no delay (or zero delay) will cause an event after delta delay, which means that the event happens only when all of the currently active processes have finished executing (i.e. after one simulation cycle).
The default delay mode ( inertial ) means that pulses shorter than the delay (or the reject period if specified) are ignored. Transport means that the assignment acts as a pure delay line.
VHDL'93 defines the keyword unaffected which indicates a choice where the signal is not given a new assignment. This is roughly equivalent to the use of the null statement within case (see Examples).
- Delays are usually ignored by synthesis tool.
Aggregate , Concurrent statement , Sequential statement , Signal , Variable assignment
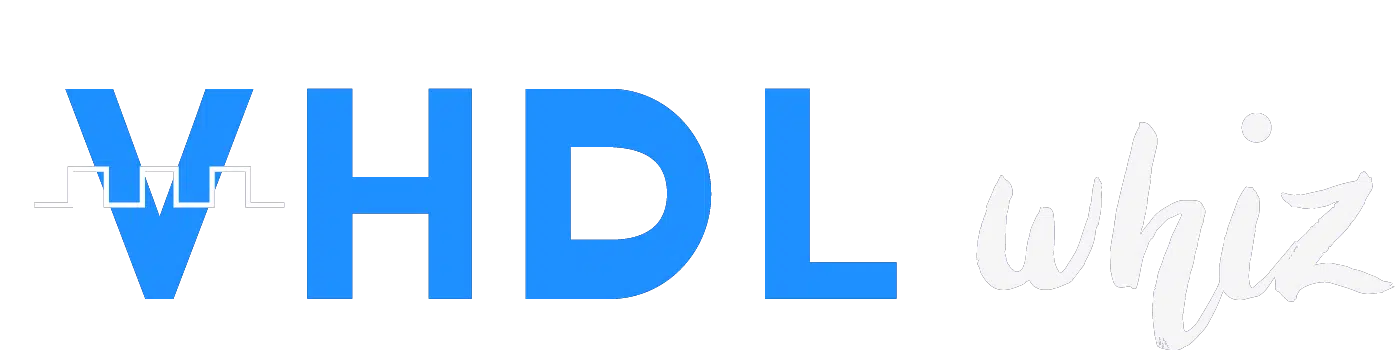
How a signal is different from a variable in VHDL
In the previous tutorial we learned how to declare a variable in a process. Variables are good for creating algorithms within a process, but they are not accessible to the outside world. If a scope of a variable is only within a single process, how can it interact with any other logic? The solution for this is a signal .
Signals are declared between the architecture <architecture_name> of <entity_name> is line and the begin statements in the VHDL file. This is called the declarative part of the architecture.
This blog post is part of the Basic VHDL Tutorials series.
The syntax for declaring a signal is:
A signal may optionally be declared with an initial value:
In this video tutorial we learn how to declare a signal. We will also learn the main difference between a variable and a signal:
The final code we created in this tutorial:
The output to the simulator console when we pressed the run button in ModelSim:
Need the Questa/ModelSim project files?
Let me send you a Zip with everything you need to get started in 30 seconds
By submitting, you consent to receive marketing emails from VHDLwhiz (unsubscribe anytime).
We created a signal and a variable with the same initial value of 0. In our process we treated them in the exact same way, yet the printouts reveal that they behaved differently. First we saw that the assignment to a variable and a signal has a different notation in VHDL. Variable assignment uses the := operator while signal assignment uses the <= operator.
MyVariable behaves as one would expect a variable to behave. In the first iteration of the loop it is incremented to 1, and then to 2. The last printout from the first iteration shows that its value is still 2, as we would expect.
MySignal behaves slightly different. The first +1 increment doesn’t seem to have any effect. The printout reveals that its value is still 0, the initial value. The same is true after the second +1 increment. Now the value of MyVariable is 2, but the value of MySignal is still 0. After wait for 10 ns; the third printout shows that the value of MySignal is now 1. The subsequent printouts follow this pattern as well.
What is this sorcery? I will give you a clue, the wait for 10 ns; has something to do with it. Signals are only updated when a process is paused. Our process pauses only one place, at wait for 10 ns; . Therefore, the signal value changes only every time this line is hit. The 10 nanoseconds is an arbitrary value, it could be anything, even 0 nanoseconds. Try it!
Another important observation is that event though the signal was incremented twice before the wait , its value only incremented once. This is because when assigning to a signal in a process, the last assignment “wins”. The <= operator only schedules a new value onto the signal, it doesn’t change until the wait . Therefore, at the second increment of MySignal , 1 is added to its old value. When it is incremented again, the first increment is completely lost.
Get free access to the Basic VHDL Course
Download the course material and get started.
You will receive a Zip with exercises for the 23 video lessons as VHDL files where you fill in the blanks, code answers, and a link to the course.
- A variable can be used within one process while signals have a broader scope
- Variable assignment is effective immediately while signals are updated only when a process pauses
- If a signal is assigned to several times without a wait , the last assignment “wins”
Go to the next tutorial »
I’m from Norway, but I live in Bangkok, Thailand. Before I started VHDLwhiz, I worked as an FPGA engineer in the defense industry. I earned my master’s degree in informatics at the University of Oslo.
Similar Posts
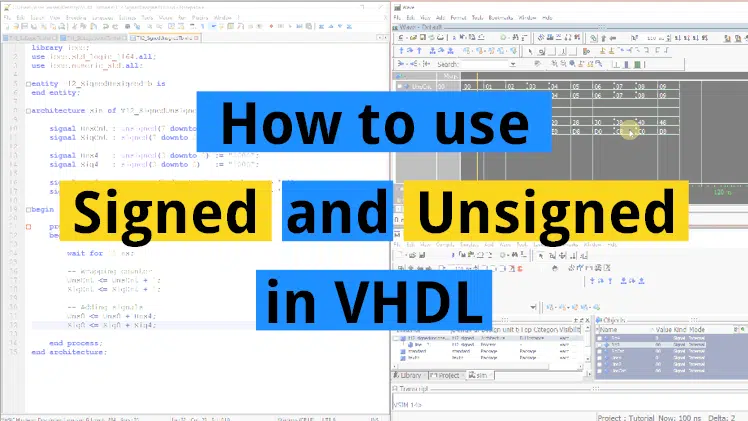
How to use Signed and Unsigned in VHDL
The signed and unsigned types in VHDL are bit vectors, just like the std_logic_vector type. The difference is that while the std_logic_vector is great for implementing data buses, it’s useless for performing arithmetic operations. If you try to add any number to a std_logic_vector type, ModelSim will produce the compilation error: No feasible entries for…
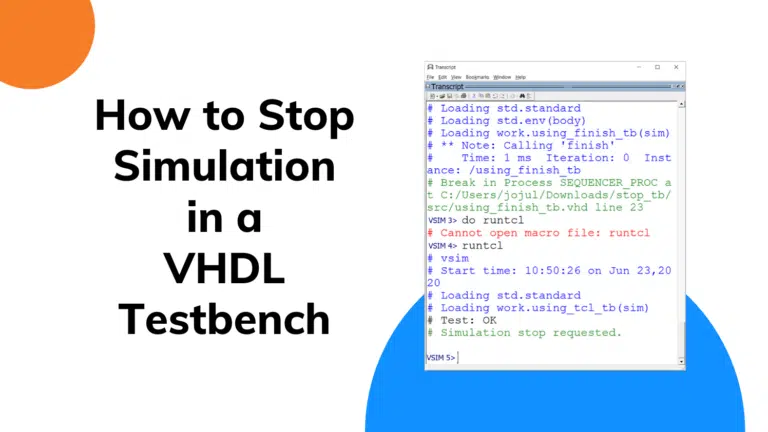
How to stop simulation in a VHDL testbench
How do you stop the VHDL simulator when the simulation is complete? There are several ways to do that. In this article, we will examine the most common ways to end a successful testbench run. The VHDL code presented here is universal, and it should work in any capable VHDL simulator. For the methods involving…
How to create a linked list in VHDL
The linked list is a dynamic data structure. A linked list can be used when the total number of elements is not known in advance. It grows and shrinks in memory, relative to the number of items it contains. Linked lists are most conveniently implemented using classes in an object-oriented programming language. VHDL has some…
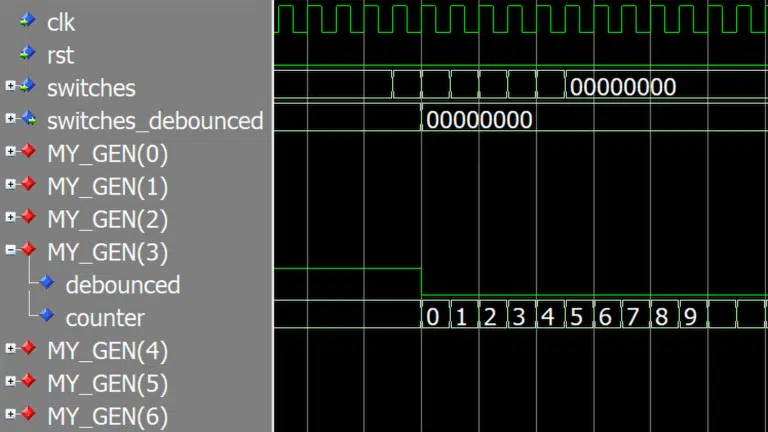
Generate statement debouncer example
The generate statement in VHDL can automatically duplicate a block of code to closures with identical signals, processes, and instances. It’s a for loop for the architecture region that can create chained processes or module instances.
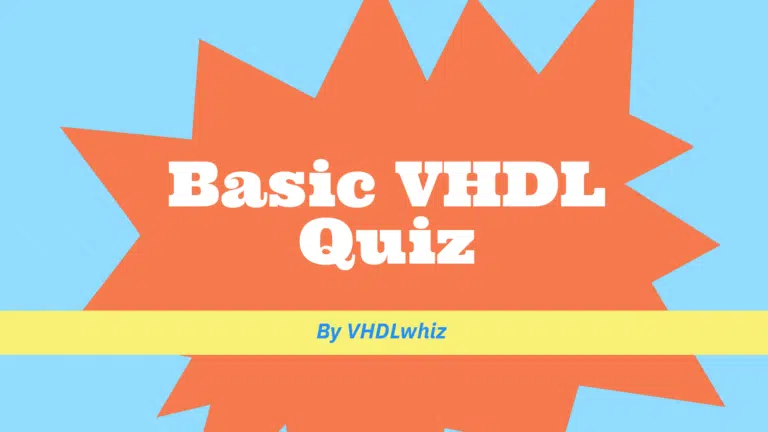
Basic VHDL quiz
Have fun and learn from this VHDL and FPGA design quiz with 28 questions for beginners and intermediate learners in random order.
All questions include an explanation for the correct answer that will be shown after you make your selection.
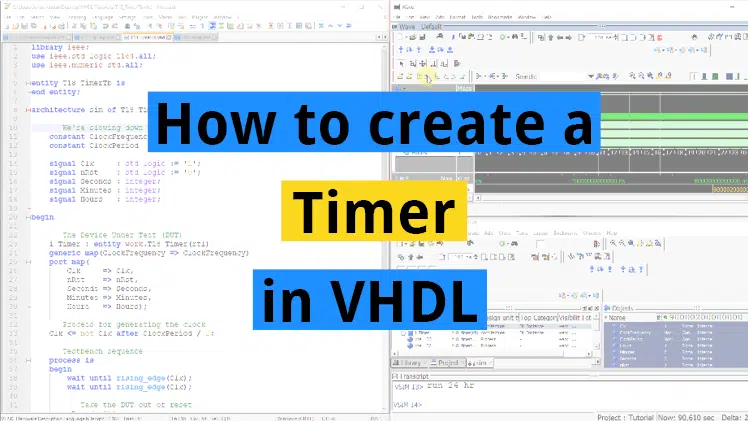
How to create a timer in VHDL
In earlier tutorials we have used the wait for statement to delay time in simulation. But what about production modules? The wait for statement cannot be used for that. That only works in simulation because we can’t just tell the electrons in a circuit to pause for a given time. So how can we keep…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Notify me of replies to my comment via email
Stack Exchange Network
Stack Exchange network consists of 183 Q&A communities including Stack Overflow , the largest, most trusted online community for developers to learn, share their knowledge, and build their careers.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Signal assignment type
What is the meaning of "combinational assignment" and "registered assignment" to signals? In particular what are the differences between these two types of assignments?
- state-machines
2 Answers 2
Essentially, the difference boils down to whether the signal gets assigned on a clock edge or not.
Combinational code, like A <= B + 1 would have A being assigned B+1 "immediately," whereas
would result in A being assigned B+1 only on a rising clock edge. With code like this, other blocks can use the value of A being guaranteed that its value will be stable and unchanging after a clock edge.
Registers, or clock gating in general I suppose, are really what make designs of any complication possible. You create a pipeline of operations by putting a register at the border between operations. For example, the input operands to an ALU must be stable - in a register- so that the ALU can execute properly, and the result of the ALU's execution should be in a register so that whatever block uses it does not 'see' the changing values inside the ALU as the calculations take place, but only the stable last result.
- \$\begingroup\$ Question: Is a transparent latch considered combinatorial or registered? \$\endgroup\$ – The Photon Commented May 14, 2012 at 4:52
- \$\begingroup\$ Yes and no - the input to a transparent latch propagates directly to the output, not on a clock edge. But the addition of an enable signal could make the latch be controlled on a clock edge, ie, opaque. \$\endgroup\$ – Kevin H Commented May 14, 2012 at 16:17
Assignments in VHDL are neighter specified as registered or combinatorial. In VHDL the actual assignment type (the type of RTL logic generated) is just inferred.
Registers in VHDL are created explicitly by assigning a signal on a clock edge, though just because a process has a clock it does not mean all signals in that block will be assigned on every edge.
Also registers can be inferred without a clock, if some path through a process does not assign a signal, then VHDL assumes you meant to latch that signal between successive passes. This is called an inferred latch (and should be avoided).
VHDL does not know about the technology that you are going to be using. It does not know whether your synthisis engine can generate T,D,JK,SR, or any other sort of latch. For that reason it just suggests a latch, it is up to the synthisis enging to decide which latch fits the bill or if it is simply impossible. Similarlay the fitter might say that a particular latch requested by the synthisis enging is not available or there are no enough of them.

Your Answer
Sign up or log in, post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
Not the answer you're looking for? Browse other questions tagged vhdl signal state-machines or ask your own question .
- The Overflow Blog
- The hidden cost of speed
- The creator of Jenkins discusses CI/CD and balancing business with open source
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
Hot Network Questions
- Matrix Multiplication & Addition
- 99 camaro overheating
- An instructor is being added to co-teach a course for questionable reasons, against the course author's wishes—what can be done?
- Could an empire rise by economic power?
- Confusion about time dilation
- Why does this theta function value yield such a good Riemann sum approximation?
- Deleting all files but some on Mac in Terminal
- Are all citizens of Saudi Arabia "considered Muslims by the state"?
- What other marketable uses are there for Starship if Mars colonization falls through?
- Nightmare Budget Bathroom Reno
- Is it safe to install programs other than with a distro's package manager?
- quantulum abest, quo minus .
- Largest number possible with +, -, ÷
- Trill with “no turn” in Lilypond
- How Does This Antique Television Circuit Work?
- Light switch that is flush or recessed (next to fridge door)
- If a Palestinian converts to Judaism, can they get Israeli citizenship?
- How should I tell my manager that he could delay my retirement with a raise?
- How can I play MechWarrior 2?
- When can the cat and mouse meet?
- What was the first "Star Trek" style teleporter in SF?
- Referencing an other tikzpicture without overlay
- What's the difference? lie down vs lie
- Convert 8 Bit brainfuck to 1 bit Brainfuck / Boolfuck
- Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers
- Advertising & Talent Reach devs & technologists worldwide about your product, service or employer brand
- OverflowAI GenAI features for Teams
- OverflowAPI Train & fine-tune LLMs
- Labs The future of collective knowledge sharing
- About the company Visit the blog
Collectives™ on Stack Overflow
Find centralized, trusted content and collaborate around the technologies you use most.
Q&A for work
Connect and share knowledge within a single location that is structured and easy to search.
Get early access and see previews of new features.
VHDL signal assignment within a process not behaving correctly?
Here's the code I'm running
You would expect that res2 would always use the previous value of sig_s1 , but in simulation it's working with the updated ones, as if this was a sequential code. What gives?
Here's the waveform https://i.sstatic.net/GSXpc.jpg (res1 is the result if I used variable instead of the signal for sig_s1). I don't know how benchmarks work.
- 1 show the full code with testbench please. if d1,d2 and d3 are all changing at the same time, then res2 should be using the old value. – vipin Commented Nov 5, 2017 at 18:18
- 5 Signal assignment is behaving correctly, but there's a mistaxe in the process sensitivity list. – user1818839 Commented Nov 5, 2017 at 18:24
- 1 Which signal in a right hand side of an assignment is not present in the process sensitivity list? – user1155120 Commented Nov 5, 2017 at 18:45
- 2 I looked at it. As Vipin says, show the full code and testbench too, not just a code snippet. – user1818839 Commented Nov 5, 2017 at 19:45
- 1 The edits don't help, especially since the signal names don't all match the waveform. What gives? You need to write a testbench and learn how to simulate properly, that's what gives. Once you can make this question an minimal reproducible example someone can help you, if you haven't figured it out in the process. – user1818839 Commented Nov 6, 2017 at 7:58
What gives is that signals do not get updated until all the processes have suspended.
Suppose there is a change (an event ) on one or more of d1 , d2 or d3 . They are in the sensitivity list of the process, so the process starts executing.
1) First this line gets executed: sig_s1 <= d1 and d2; . This is a line with a signal assignment in it ( <= ). The effect of executing any line with a signal assignment in it is, if the value of that signal is to change, to schedule that change for sometime in the future. If no delay is specified (which is the case here), then that future time is the next iteration of the simulation, which will occur once all the processes have finished executing (have suspended).
2) Then this line gets executed res2 <= sig_s1 xor d3; . Since, the effect of executing the previous line was to schedule a change on sig_s1 for the future, the value of signal sig_s1 has yet to change when this line is executed . That is really, really important. Hence the bold type. Consequently, the previous value of signal sig_s1 will be used to evaluate the expression sig_s1 xor d3 . If this results in a change to signal res2 then that change will be scheduled for the next simulation iteration, too.
There are various solutions to your problem. The simplest is to add signal sig_s1 to the sensitivity list:
Then once any change on signal sig_s1 is actioned, the process is executed again (because signal sig_s1 is now in the sensitivity list. This line will be executed first sig_s1 <= d1 and d2 and, assuming signals d1 and d2 haven't already changed again, there will be no change to signal sig_s1 . Then the line res2 <= sig_s1 xor d3 will be executed immediately afterwards. Signal sig_s1 will now have its new value and so the value of signal res2 will be updated (on the next simulation iteration, as before).
However, I recommend you don't do that. You don't need a process at all with simple expressions like this. It would be much better to do away with the process and just use concurrent signal assignments , like this:
Each concurrent signal assignment (as the name suggests) runs concurrently and so is a process in itself. (So, now there are two concurrent processes). With a concurrent signal assignment, you get a sensitivity list for free. So, there is no possibility of missing a signal out of the sensitivity list. Not only that, it takes up less space on the page, which means that the reader can see more of the code at once and hence get a better understanding*.
Always implement simple combinational logic like this as concurrent signal assignments, not as a process.
*I'm a prolific commenter, which rather undoes that advantage. Ho hum.

Your Answer
Reminder: Answers generated by artificial intelligence tools are not allowed on Stack Overflow. Learn more
Sign up or log in
Post as a guest.
Required, but never shown
By clicking “Post Your Answer”, you agree to our terms of service and acknowledge you have read our privacy policy .
- The Overflow Blog
- The hidden cost of speed
- The creator of Jenkins discusses CI/CD and balancing business with open source
- Featured on Meta
- Announcing a change to the data-dump process
- Bringing clarity to status tag usage on meta sites
- What does a new user need in a homepage experience on Stack Overflow?
- Feedback requested: How do you use tag hover descriptions for curating and do...
- Staging Ground Reviewer Motivation
Hot Network Questions
- Can Christian Saudi Nationals visit Mecca?
- Quantum Gravity is non-renormalizable...So what?
- Cohomological range of a perverse sheaf
- Is there a way to do a PhD such that you get a broad view of a field or subfield as a whole?
- quantulum abest, quo minus .
- Trill with “no turn” in Lilypond
- Matrix Multiplication & Addition
- Does a party have to wait 1d4 hours to start a Short Rest if no healing is available and an ally is only stabilized?
- Why is Haji Najibullah's case being heard in federal court in the Southern District of NY?
- How do I apologize to a lecturer who told me not to ever call him again?
- Why is a USB memory stick getting hotter when connected to USB-3 (compared to USB-2)?
- Why are poverty definitions not based off a person's access to necessities rather than a fixed number?
- Maximisation of product
- What's the difference? lie down vs lie
- Are all citizens of Saudi Arabia "considered Muslims by the state"?
- Why doesn’t dust interfere with the adhesion of geckos’ feet?
- What's the benefit or drawback of being Small?
- Is it possible to recover from a graveyard spiral?
- Dirichlet Series that fail to be L-functions
- How do I safely download and run an older version of software for testing without interfering with the currently installed version?
- Does it make sense for the governments of my world to genetically engineer soldiers?
- Star Trek: The Next Generation episode that talks about life and death
- What's "the archetypal book" called?
- Fusion September 2024: Where are we with respect to "engineering break even"?

COMMENTS
The VHDL code shown below uses one of the logical operators to implement this basic circuit. and_out <= a and b; Although this code is simple, there are a couple of important concepts to consider. The first of these is the VHDL assignment operator (<=) which must be used for all signals.
Variables and Signals in VHDL appears to be very similar. They can both be used to hold any type of data assigned to them. The most obvious difference is that variables use the := assignment symbol whereas signals use the <= assignment symbol. However the differences are more significant than this and must be clearly understood to know when to ...
With / Select. The most specific way to do this is with as selected signal assignment. Based on several possible values of a, you assign a value to b. No redundancy in the code here. The official name for this VHDL with/select assignment is the selected signal assignment. with a select b <= "1000" when "00", "0100" when "01", "0010" when "10 ...
Conditional Signal Assignment or the "When/Else" Statement. The "when/else" statement is another way to describe the concurrent signal assignments similar to those in Examples 1 and 2. Since the syntax of this type of signal assignment is quite descriptive, let's first see the VHDL code of a one-bit 4-to-1 multiplexer using the ...
Select Statement - VHDL Example Assigning signals using Selected signal assignment. Select statements are used to assign signals in VHDL. They can only be used in combinational code outside of a process. A selected signal assignment is a clear way of assigning a signal based on a specific list of combinations for one input signal.
The Inside_process and Outside_process versions behave differently. If both designs work, it is mostly out of luck, because in this case Out_signal simply lags half a clock cycle when declared inside the process. Out_signal is assigned when the process triggers, which in this case occurs on rising and falling edges of clk.
Any variable which does not assign its value to a signal, but only to other variables, is a perfectly acceptable "wire". My understanding of the whole subject is this: A signal assignment inside a process will disregard other signal assignments made in the same process "instantiation".
A VHDL description has two domains: a sequential domain and a concurrent domain. The sequential ... sequential signal assignment, variable assignment, if statement, case statement, loop statements (loop, while loop, for, next, exit), and the sequential assert statement. Besides these statements, other sequential statements are the pro-
A Signal assignment statement can appear inside a process (sequential statement) or directly in an architecture (concurrent statement). The target signal can be either a name (simple, selected, indexed, or slice) or an aggregate. A signal assignment with no delay (or zero delay) will cause an event after delta delay, which means that the event ...
First we saw that the assignment to a variable and a signal has a different notation in VHDL. Variable assignment uses the := operator while signal assignment uses the <= operator. MyVariable behaves as one would expect a variable to behave. In the first iteration of the loop it is incremented to 1, and then to 2.
2. When updated. local variable is immediately updated whe. thevariable assignment statement is executed.A signal. ssignment statement updates the signal driver. The new value of the. gn. ent between variables statement and signals3. Variables are cheaper to implement in VHDL simulatio. since the evaluation of.
VHDL-93 defines an unaffected keyword, which indicates a condition when a signal is not given a new assignment: label: signal = expression_1 when condition_1 else expression_2 when condition_2 else unaffected ; The keywords inertial and reject may also be used in a conditional signal assignment.
A signal in VHDL represents a physical interconnect. You can see that in this example, signals feed the first process on the left, which could represent something like a MUX. ... Signal assignments are defined with the less than equal to symbol as shown in these examples. The first example shows all eight bits being assigned at once. In this ...
1. Assignments in VHDL are neighter specified as registered or combinatorial. In VHDL the actual assignment type (the type of RTL logic generated) is just inferred. Registers in VHDL are created explicitly by assigning a signal on a clock edge, though just because a process has a clock it does not mean all signals in that block will be assigned ...
In VHDL there are two assignment symbols: <= Assignment of Signals. := Assignment of Variables and Signal Initialization. Either of these assignment statements can be said out loud as the word "gets". So for example in the assignment: test <= input_1; You could say out loud, "The signal test gets (assigned the value from) input_1.".
As such It's scope is limited. There tends to be a less direct relationship to synthesized hardware. Variables also get a value immediately, whereas signals don't. the following two processes have the same effect: signal IP, NEXTP : STD_LOGIC_VECTOR(0 to 5); process (CLK) Variable TEMP : STD_LOGIC_VECTOR(0 to 5);
1. The signals should be driven from the same process : multiple drivers would interfere with each other.. See Is process in VHDL reentrant? on signal assignment semantics. now you can see there is need for some delay (even just 2 delta cycles, if the logic calculating VALUE is just a simple signal assignment) between the X and LED0 assignments.
1. As user1155120 says, in VHDL the width of the right hand side has to match the width of the left hand side of an assignment operator (<= or :=). So, you could use the literal that corresponds to a std_logic_vector, which is a string: signal Qout: Std_Logic_Vector (4 downto 0) := "00001"; (a string literal in VHDL is enclosed within double ...
architecture arch of func signal sig_s1: std_logic; begin sig_s1 <= d1 and d2; res2 <= sig_s1 xor d3; end arch; Each concurrent signal assignment (as the name suggests) runs concurrently and so is a process in itself. (So, now there are two concurrent processes). With a concurrent signal assignment, you get a sensitivity list for free.